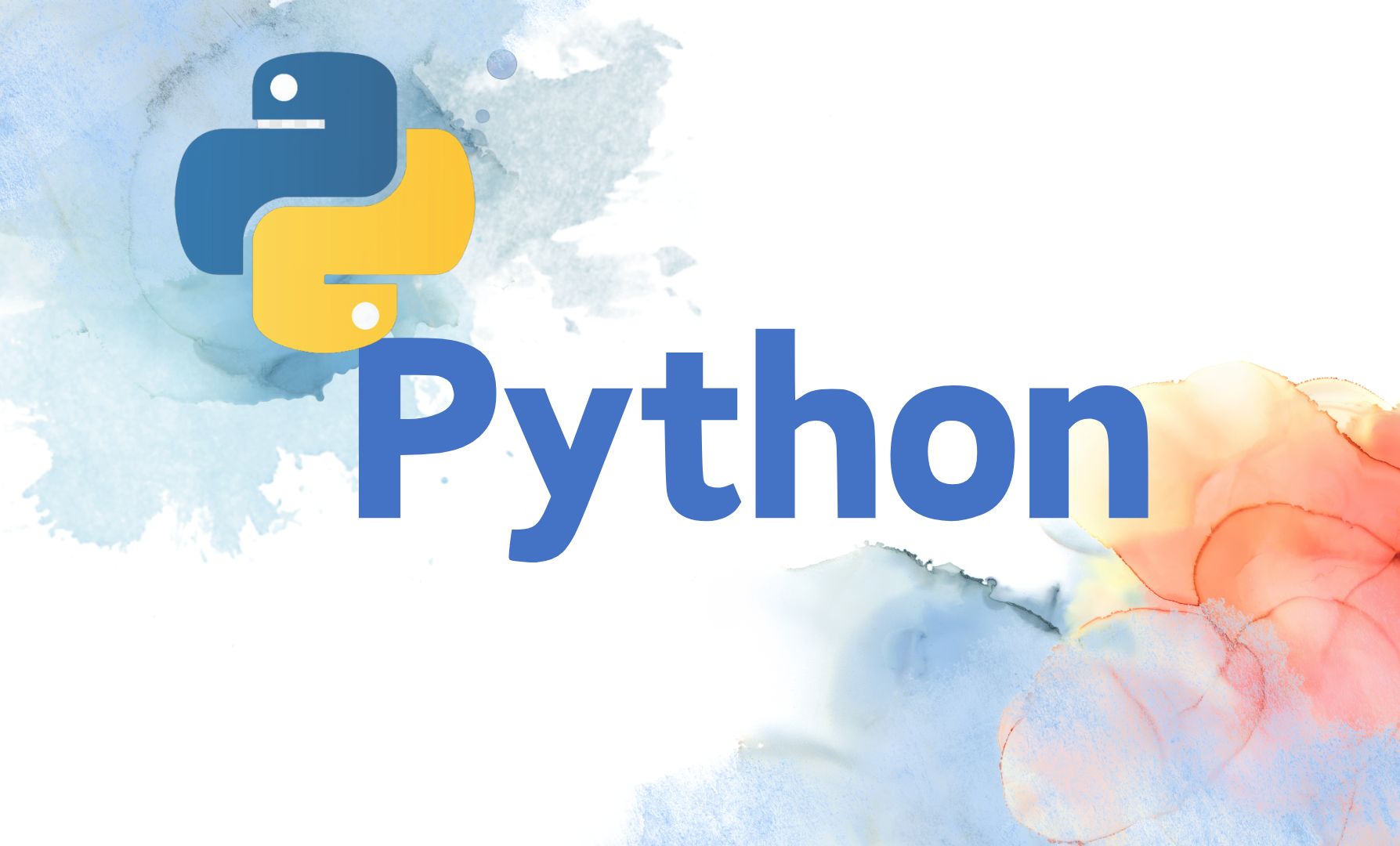
Python操作Excel之二
在python中操作excel会使用如下代码:
df = pd.read_excel(file_stream)
data = df.to_dict(orient='records') # 输出形如:[{'Name': 'Alice', 'Age': 25}, {'Name': 'Bob', 'Age': 30}]
其中orient='records'是什么意思呢?
以下是 orient
支持的所有模式及其效果:
1. orient='dict'
(默认)
结构:字典的键是列名,值是对应列的 数据列表。
示例:
{ "列名1": [值1, 值3, ...], "列名2": [值2, 值4, ...], ... }
2. orient='list'
结构:与
orient='dict'
类似,但外层用列表包装。示例:
[ {"列名1": 值1, "列名2": 值2, ...}, {"列名1": 值3, "列名2": 值4, ...}, ... ]
3. orient='split'
结构:将数据拆分为键
index
、columns
和data
。示例:
{ "index": [行索引1, 行索引2, ...], "columns": ["列名1", "列名2", ...], "data": [ [值1, 值2, ...], # 第1行 [值3, 值4, ...], # 第2行 ... ] }
4. orient='index'
结构:字典的键是行索引,值是每行的数据字典。
示例:
{ 行索引1: {"列名1": 值1, "列名2": 值2, ...}, 行索引2: {"列名1": 值3, "列名2": 值4, ...}, ... }
5. orient='tight'
(Pandas 1.4+)
结构:类似
orient='split'
,但包含更多元数据。示例:
{ "index": [行索引1, 行索引2, ...], "columns": ["列名1", "列名2", ...], "data": [[值1, 值2, ...], [值3, 值4, ...], ...], "index_names": [索引名], "column_names": [列名] }
对比示例
假设有一个 DataFrame:
import pandas as pd
df = pd.DataFrame({
"Name": ["Alice", "Bob"],
"Age": [25, 30]
})
使用 orient='records'
print(df.to_dict(orient='records'))
# 输出:
# [{'Name': 'Alice', 'Age': 25}, {'Name': 'Bob', 'Age': 30}]
使用 orient='dict'
print(df.to_dict(orient='dict'))
# 输出:
# {'Name': {0: 'Alice', 1: 'Bob'}, 'Age': {0: 25, 1: 30}}
如何选择 orient
参数?
API 响应:选
'records'
(直接对应 JSON 数组)。按列处理数据:选
'dict'
或'list'
。需要行索引信息:选
'index'
或'split'
。兼容其他工具:根据目标格式要求选择(如某些库需要
'split'
)。
总结
orient='records'
生成的是 按行组织的字典列表,适合直接转换为 JSON 数组。其他
orient
参数提供了灵活的数据结构适配,可根据具体需求选择。