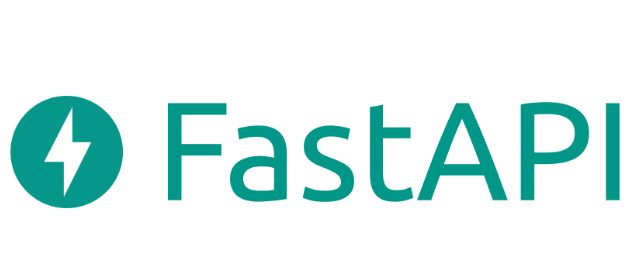
FastAPI学习指南
学习FastAPI可以通过以下分步指南进行,涵盖从基础到进阶的关键知识点:
1. 安装与环境配置
安装Python 3.7+:确保Python版本符合要求。
安装FastAPI和Uvicorn:
pip install fastapi uvicorn
2. 创建第一个FastAPI应用
新建文件
main.py
,写入以下代码:from fastapi import FastAPI app = FastAPI() @app.get("/") async def root(): return {"message": "Hello World"}
运行应用:
uvicorn main:app --reload
访问
http://localhost:8000
查看返回的JSON消息。访问
http://localhost:8000/docs
查看Swagger文档。
3. 路径参数与查询参数
路径参数(URL中的动态部分):
@app.get("/items/{item_id}") async def read_item(item_id: int): return {"item_id": item_id}
查询参数(URL中的键值对):
@app.get("/items/") async def read_items(skip: int = 0, limit: int = 10): return {"skip": skip, "limit": limit}
访问
http://localhost:8000/items/5
返回{"item_id":5}
访问
http://localhost:8000/items/?skip=2&limit=3
返回{"skip":2,"limit":3}
4. 请求体与Pydantic模型
定义数据模型:
from pydantic import BaseModel class Item(BaseModel): name: str description: str | None = None price: float
使用模型处理POST请求:
@app.post("/items/") async def create_item(item: Item): return item
在Swagger UI中测试,输入JSON数据提交。
5. 响应模型与数据过滤
指定返回数据的结构:
@app.post("/items/", response_model=Item) async def create_item(item: Item): return item
即使输入包含额外字段,响应模型会过滤掉未定义的字段。
6. 异步支持
使用
async def
定义异步函数:@app.get("/async-example") async def async_read(): await some_async_operation() return {"message": "Async done"}
7. 中间件与CORS
添加CORS中间件:
from fastapi.middleware.cors import CORSMiddleware app.add_middleware( CORSMiddleware, allow_origins=["*"], # 允许所有源(生产环境应限制) allow_methods=["*"], allow_headers=["*"], )
8. 错误处理
抛出HTTP异常:
from fastapi import HTTPException @app.get("/items/{item_id}") async def read_item(item_id: int): if item_id not in items: raise HTTPException(status_code=404, detail="Item not found") return {"item": items[item_id]}
9. 依赖注入
创建依赖函数:
from fastapi import Depends async def common_params(q: str | None = None): return {"q": q} @app.get("/items/") async def read_items(commons: dict = Depends(common_params)): return commons
10. 文件上传
处理文件上传:
from fastapi import UploadFile, File @app.post("/uploadfile/") async def upload_file(file: UploadFile = File(...)): return {"filename": file.filename}
11. 安全与认证
OAuth2与JWT示例:
from fastapi.security import OAuth2PasswordBearer oauth2_scheme = OAuth2PasswordBearer(tokenUrl="token") @app.get("/users/me") async def read_current_user(token: str = Depends(oauth2_scheme)): return {"token": token}
12. 数据库集成(以SQLAlchemy为例)
安装SQLAlchemy:
pip install sqlalchemy
定义模型与数据库会话:
from sqlalchemy import create_engine, Column, Integer, String from sqlalchemy.ext.declarative import declarative_base from sqlalchemy.orm import sessionmaker SQLALCHEMY_DATABASE_URL = "sqlite:///./test.db" engine = create_engine(SQLALCHEMY_DATABASE_URL) SessionLocal = sessionmaker(autocommit=False, autoflush=False, bind=engine) Base = declarative_base() class User(Base): __tablename__ = "users" id = Column(Integer, primary_key=True, index=True) name = Column(String)
13. 部署到生产环境
使用Uvicorn生产配置:
uvicorn main:app --host 0.0.0.0 --port 80 --workers 4
Docker示例:
FROM python:3.9 WORKDIR /app COPY requirements.txt . RUN pip install -r requirements.txt COPY . . CMD ["uvicorn", "main:app", "--host", "0.0.0.0", "--port", "80"]
14. 进一步学习资源
官方文档:FastAPI官方文档
GitHub仓库:FastAPI GitHub
教程视频:B站上的FastAPI教程系列。
通过逐步实践每个部分,并结合实际项目(如构建一个待办事项API或用户管理系统),可以深入掌握FastAPI的使用。